How to Implement WellSaid Labs TTS API In Laravel?
WellSaid Labs text-to-speech API service allows you to augment existing applications with a life-like synthetic voice.
Step 1: First, we have to create an account on https://wellsaidlabs.com website.
Step 2: After Sign-up, go to the https://developer.wellsaidlabs.com/#!/ link and you have to log in with your account.
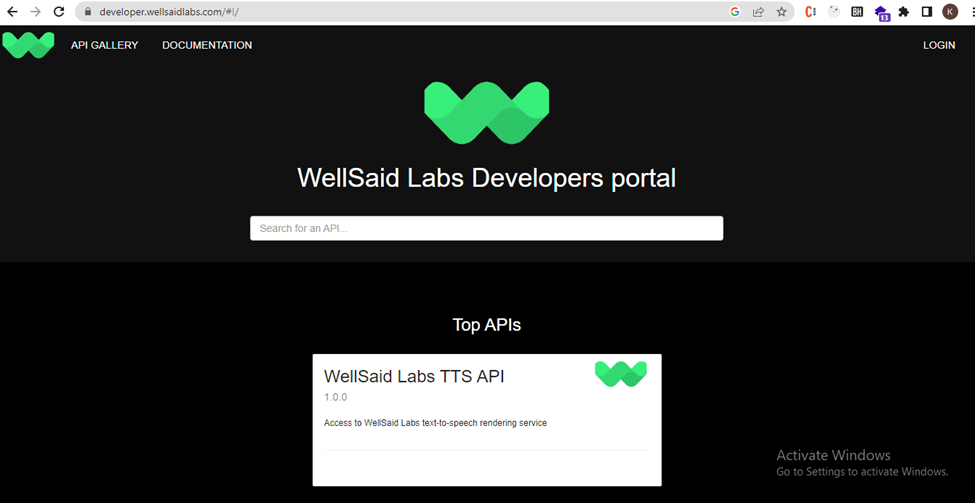
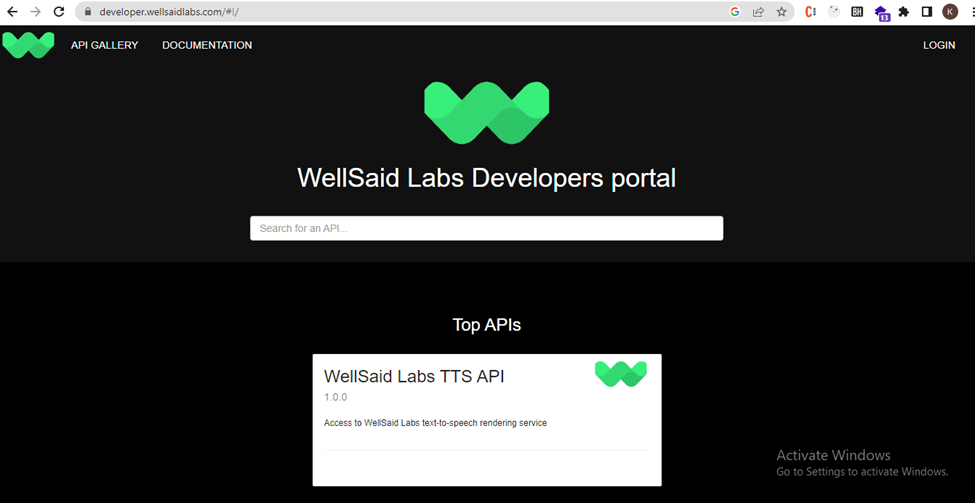
Step 3: Click on the below WellSaid Labs TTS API’s section:
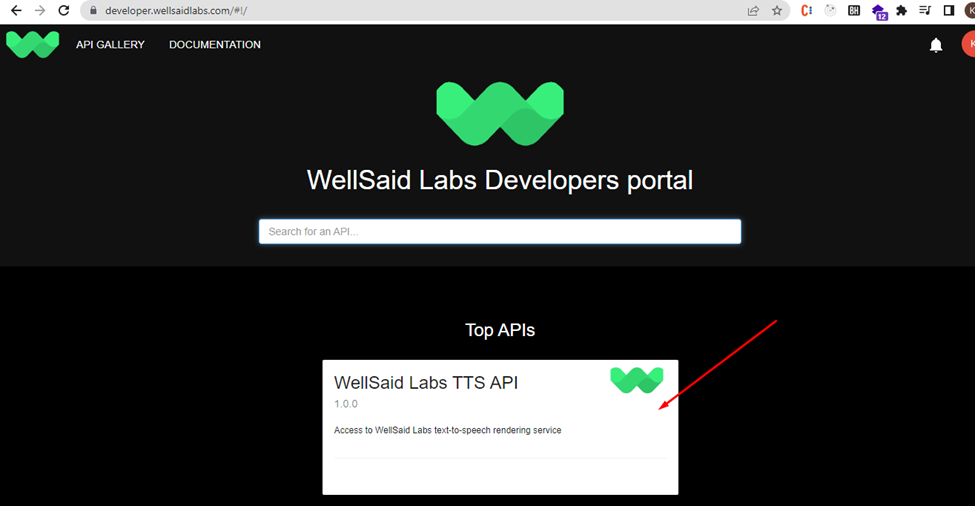
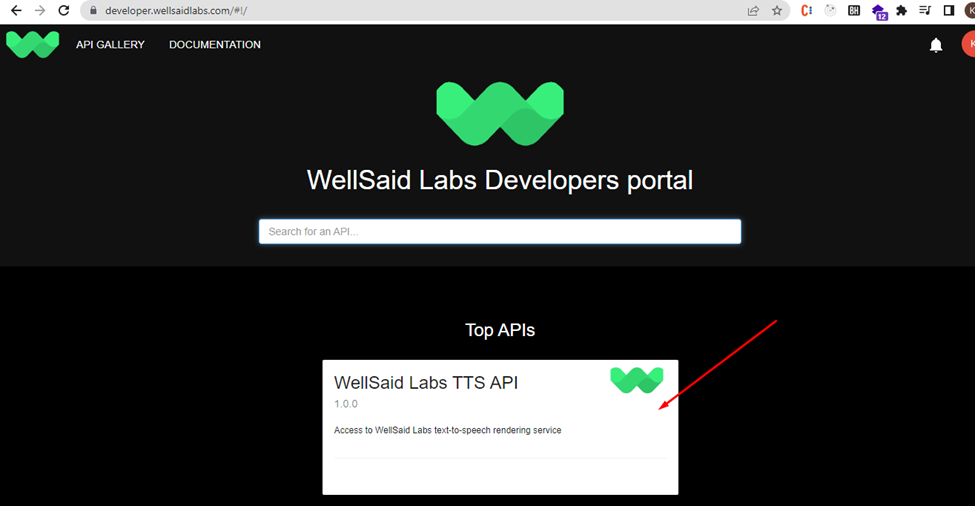
Step 4: The next step in using this service is requesting access via the Subscribe
link on our developer portal. Once approved, you will have access to the WellSaid Labs TTS API through the use of an API key.
Step 5: You can use all or any of these available speakers with the given id from the WellSaid Labs TTS API’s details page.
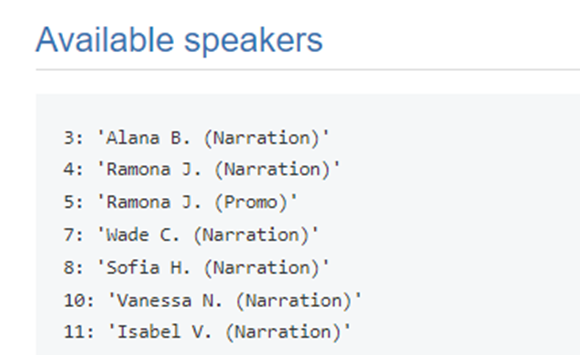
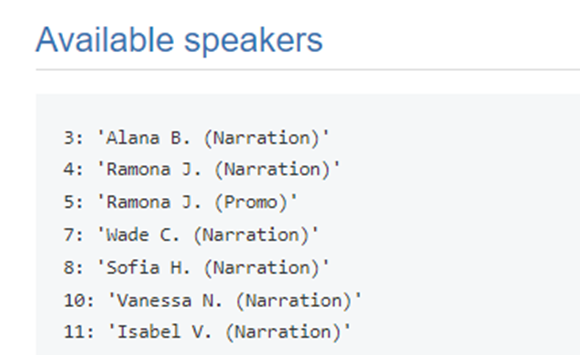
..and more
Step 6: After getting the API key, you can use as per the given example:
Index.blade.php
1234567891011121314151617181920212223242526272829303132333435
<html><body><div style="text-align: center;"><select name="user_name" id="user_name"> <option value="3">Alana B.</option> <option value="4" selected="">Ramona J.</option> ..... <option value="95">Greg G.</option> </select><br><br> <textarea rows="8" name="decription" id="decription" placeholder="enter text here..."></textarea><br> <div id="audio_output"></div><br> <input type="button" class="get-clip" name="audio_clip" value="Get Clip"></div></body></html><script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.js" type="text/javascript"></script><script> $(document).ready(function(){ $(document).on('click','.get-clip',function() { var id = $('#user_name').val(); var text = $('#decription').val(); $.ajax({ type:'post', url:"{{ route('wave.audio-clip') }}", data:{'text': text, 'speaker_id' : id, '_token': "{{ csrf_token() }}"}, dataType: 'html', success:function(data){$('#audio_output').html('<audio id="audioclip" controls=""><source src="'+data+'" type="audio/mpeg"></audio>'); } }); });}); </script>
web.php
1234
Route::get('audio-test', '\Wave\Http\Controllers\AudioTestController@audio'); Route::post('audio', '\Wave\Http\Controllers\AudioTestController@audio_clip')>name('wave.audio-clip');
AudioTestController.php
123456789101112131415161718192021222324252627282930313233343536373839404142
<?php namespace Wave\Http\Controllers;use App\Http\Controllers\Controller;use Illuminate\Http\Request; class AudioTestController extends Controller{ public function audio(){ return view('index'); } public function audio_clip(Request $request){ $ch = curl_init(); $postData = [ 'text' => $request->text, 'speaker_id' => $request->speaker_id ]; curl_setopt($ch, CURLOPT_URL, 'https://api.wellsaidlabs.com/v1/tts/stream'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($postData));$headers = array(); $headers[] = 'Your API Key'; $headers[] = 'Accept: audio/mpeg'; $headers[] = 'Content-Type: application/json'; curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); $result = curl_exec($ch); if (curl_errno($ch)) { echo 'Error:' . curl_error($ch); } $filename = time(); $file_path = public_path("$filename.mp3"); file_put_contents($file_path, $result); curl_close($ch); echo env('APP_URL').$filename.'.mp3'; }
Step 7: Run the above Laravel example.